- On Eclipse, click File->New->Other->Anroid Project
- Create a new Android Project with the following …
- Project name= LoanCalculator
- Target= Android 1.5
- Application name= LoanCalculator
- Package name=com.androidfun.loancalculator
- [checked] Create Activity = LoanCalculatorActivity
- Min SDK Version = 3
Create the LoanCalculator GUI
- Android UI components can be created declaratively by XML or programmatically inside the code. For now, we are using XML. The main.xml (i.e. LoanCalculator/res/layout/main.xml) defines the UI for LoanCalculatorActivity.
public class LoanCalculatorActivity extends Activity {
/** Called when the activity is first created. */
@Override public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main); }
}
- The content view of the LoanCalculatorActivity is sourced from the main.xml in ./res/layout folder by calling setContentView(...)
- We would like to have a Calculator view looks like the diagram below.
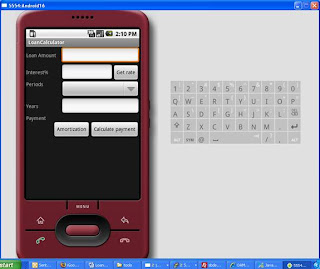
- Layout management in main.xml. The view has a vertical stack of rows of widget. The vertical stack is declared as the outer <Linearlayout> element with anroid:orientation="vertical". Each row of widgets is a <Linearlayout> element with anroid:orientation="horizaontal".
- In main.xml, we use:
- <Textview> for label
- <Edittext> for text entry field
- <Spinner> for drop down selection
- <Button> for button
Note the two Android attributes in the view declaration elements.
- android:id="@+id/annualRate" declares the ID of the widget, the widget can be referenced in Java by (EditText)findViewById(R.id.annualRate);
- android:text="@string/annualRate" reference the string value in res/values/strings.xml
Java classes
We create two Java classes ...
- LoanCalculator.java - A POJO domain object that encapsulates the loan calculator formula
- LoanCalculatorActivity - Subclasses from Android's Activity class, the controller that links the GUI and the LoanCalculator domain object.
Listing of LoanCalculatorActivity.java
LoanCalculator class walk through
onCreate() - Look up the widget declared in main.xml, create and register event listeners for buttons and initialize the default valus in the view.
initPeriodsSpinner() - Example of populating the selections with values from resource file (i.e. array.xml) to a Spinner widget.
addCalcPmtListener() - Example of creating an event listener for a button.
populateView() - Populating the value to the widgets from a domain object.
DOWNLOAD the LoanCalculator Eclipse project (LoanCalculator_1_1.zip)